Table of Contents
Description: Evaluate Boolean Binary
You are given the root
of a full binary tree with the following properties:
- Leaf nodes have either the value
0
or1
, where0
representsFalse
and1
representsTrue
. - Non-leaf nodes have either the value
2
or3
, where2
represents the booleanOR
and3
represents the booleanAND
.
The evaluation of a node is as follows:
- If the node is a leaf node, the evaluation is the value of the node, i.e.
True
orFalse
. - Otherwise, evaluate the node’s two children and apply the boolean operation of its value with the children’s evaluations.
Return the boolean result of evaluating the root
node.
A full binary tree is a binary tree where each node has either 0
or 2
children.
A leaf node is a node that has zero children.
Example 1
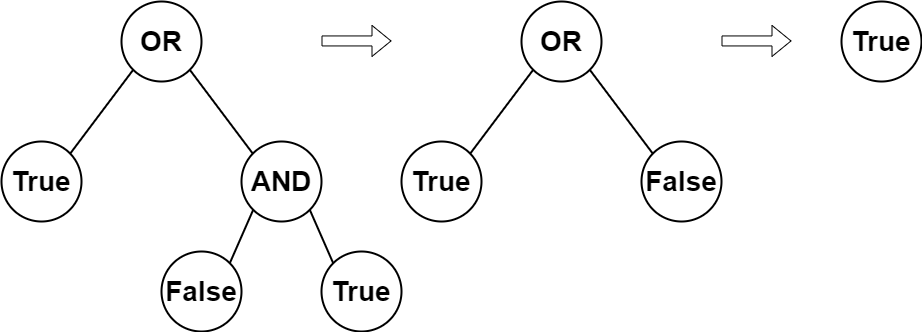
1 2 3 4 5 6 |
<strong>Input:</strong> root = [2,1,3,null,null,0,1] <strong>Output:</strong> true <strong>Explanation:</strong> The above diagram illustrates the evaluation process. The AND node evaluates to False AND True = False. The OR node evaluates to True OR False = True. The root node evaluates to True, so we return true. |
Example 2
1 2 3 |
<strong>Input:</strong> root = [0] <strong>Output:</strong> false <strong>Explanation:</strong> The root node is a leaf node and it evaluates to false, so we return false. |
Constraints
- The number of nodes in the tree is in the range
[1, 1000]
. 0 <= Node.val <= 3
- Every node has either
0
or2
children. - Leaf nodes have a value of
0
or1
. - Non-leaf nodes have a value of
2
or3
.
Solution
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 |
/** * Definition for a binary tree node. * public class TreeNode { * int val; * TreeNode left; * TreeNode right; * TreeNode() {} * TreeNode(int val) { this.val = val; } * TreeNode(int val, TreeNode left, TreeNode right) { * this.val = val; * this.left = left; * this.right = right; * } * } */ class Solution { //Post order Traversal public boolean evaluateTree(TreeNode root) { if(root==null){ return false; } if(root.left==null && root.right==null){ return root.val==0?false:true; } boolean l = evaluateTree(root.left); boolean r = evaluateTree(root.right); if(root.val==2){ return l|r; } return l&r; } } |
Time Complexity
O(n), n is the number of nodes in a Binary tree
Space Complexity
O(n), n is the number of nodes in a Binary Tree (recursion stack)