source: https://leetcode.com/problems/two-sum-bsts/
Two Sum BSTs
Description
Given the roots of two binary search trees, root1 and root2, return true if and only if there is a node in the first tree and a node in the second tree whose values sum up to a given integer target.
Example 1:
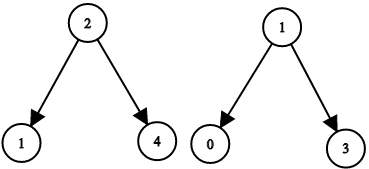
Input: root1 = [2,1,4], root2 = [1,0,3], target = 5
Output: true
Explanation: 2 and 3 sum up to 5.
Example 2:
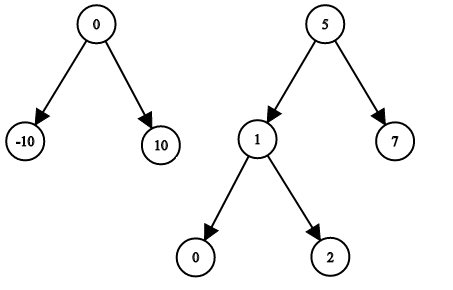
Input: root1 = [0,-10,10], root2 = [5,1,7,0,2], target = 18
Output: false
Constraints:
- The number of nodes in each tree is in the range [1, 5000].
- -109 <= Node.val, target <= 109
Solution
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
/** * Definition for a binary tree node. * public class TreeNode { * int val; * TreeNode left; * TreeNode right; * TreeNode() {} * TreeNode(int val) { this.val = val; } * TreeNode(int val, TreeNode left, TreeNode right) { * this.val = val; * this.left = left; * this.right = right; * } * } */ class Solution { public boolean twoSumBSTs(TreeNode root1, TreeNode root2, int target) { return helper(root1, root2, target); } private boolean helper(TreeNode node1, TreeNode node2, int target){ if(node1 == null || node2 == null) return false; int sum = node1.val + node2.val; if(sum == target){ return true; } boolean ans = false; if(sum < target){ ans = helper(node1, node2.right, target) || helper(node1.right, node2, target); } else { ans = helper(node1.left, node2, target) || helper(node1, node2.left, target); } return ans; } } |