You are given a tree (i.e., a connected, undirected graph that has no cycles) consisting of n
nodes numbered from 0
to n - 1
and exactly n - 1
edges
. The root of the tree is the node 0
, and each node of the tree has a label which is a lower-case character given in the string labels
(i.e., The node with the number i
has the label labels[i]
). The edges
array is given on the form edges[i] = [ai, bi]
, which means there is an edge between nodes ai
and bi
in the tree. Return an array of size n where ans[i] is the number of nodes in the subtree of the ith node, which have the same label as the node i
. A subtree of a tree T
is the tree consisting of a node in T
and all of its descendant nodes.
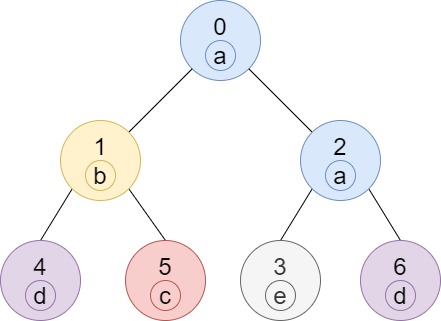
1 2 3 4 |
<strong>Input:</strong> n = 7, edges = [[0,1],[0,2],[1,4],[1,5],[2,3],[2,6]], labels = "abaedcd" <strong>Output:</strong> [2,1,1,1,1,1,1] <strong>Explanation:</strong> Node 0 has label 'a' and its sub-tree has node 2 with label 'a' as well, thus the answer is 2. Notice that any node is part of its sub-tree. Node 1 has a label 'b'. The sub-tree of node 1 contains nodes 1,4 and 5, as nodes 4 and 5 have different labels than node 1, the answer is just 1 (the node itself). |
https://leetcode.com/problems/number-of-nodes-in-the-sub-tree-with-the-same-label/description/
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 |
class Solution { public int[] countSubTrees(int n, int[][] edges, String labels) { //create a graph Map<Integer, List<Integer>> graph = new HashMap<>(); for(int[] edge: edges){ //undirected graph //add edge a->b addEdge(graph, edge[0], edge[1]); //add edge b->a addEdge(graph, edge[1], edge[0]); } //to keep track of the visited nodes Set<Integer> visited = new HashSet<>(); int[] count = new int[n]; dfs(graph, 0, -1, visited, labels, count); return count; } private int[] dfs(Map<Integer, List<Integer>> graph, int node, int parent, Set<Integer> visited, String labels, int[] count){ //to keep track the count at the current node int[] total = new int[26]; //if the node is not visited yet if(!visited.contains(node)){ if(graph.containsKey(node)){ //visit all the children of the node for(int adj: graph.get(node)){ //to prevent cycle if(adj!=parent){ int[] arr = dfs(graph, adj, node, visited, labels, count); //the count current node will be the sum of counts at the child nodes for(int i=0;i<26;i++){ total[i] = arr[i] + total[i]; } } } } //mark the node as visited visited.add(node); } char c = labels.charAt(node); //add node's self count total[c-'a']++; count[node]=total[c-'a']; return total; } //add an edge to graph - represented by an adjacency list private void addEdge(Map<Integer, List<Integer>> graph, int a, int b){ List<Integer> list = graph.getOrDefault(a, new ArrayList<>()); list.add(b); graph.put(a, list); } } |