source: https://leetcode.com/problems/check-if-it-is-a-straight-line/description/
Check If It Is a Straight Line
Table of Contents
Description
You are given an array coordinates, coordinates[i] = [x, y], where [x, y] represents the coordinate of a point. Check if these points make a straight line in the XY plane.
Example 1:
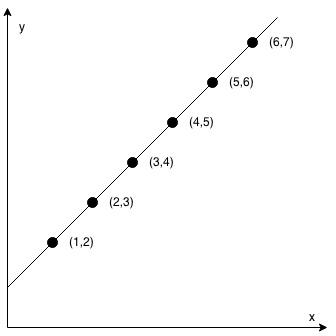
Input: coordinates = [[1,2],[2,3],[3,4],[4,5],[5,6],[6,7]]
Output: true
Example 2:
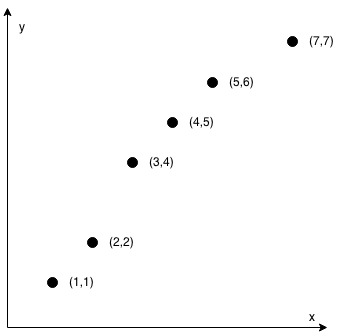
Input: coordinates = [[1,1],[2,2],[3,4],[4,5],[5,6],[7,7]]
Output: false
Constraints:
- 2 <= coordinates.length <= 1000
- coordinates[i].length == 2
- -10^4 <= coordinates[i][0], coordinates[i][1] <= 10^4
- coordinates contains no duplicate point.
Solution
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
class Solution { public boolean checkStraightLine(int[][] coordinates) { if(coordinates.length==2){ return true; } for(int i=2;i<coordinates.length;i++){ if(!check(coordinates[0][0], coordinates[0][1], coordinates[1][0], coordinates[1][1], coordinates[i][0], coordinates[i][1])){ return false; } } return true; } private boolean check(int x1, int y1, int x2, int y2, int x, int y){ //equation of a line return (y2 - y1)*x - (x2- x1)*y - (y2-y1)*x1 + (x2-x1)*y1 == 0; } } |